Introduction
Thread starvation can be a silent killer of performance in C# applications, causing frustration when your app slows down due to blocked threads. But don't worry; we're here to help you regain control! In this guide, we’ll explore three powerful techniques: Task.WhenAll, Semaphores, and Parallel.ForEachAsync. These are practical solutions to enhance thread management and restore your app's peak performance.
The Hidden Threat to Your App's Performance!
Thread starvation becomes particularly problematic with rate-limited services. Imagine your application as a busy delivery service; if too many threads (couriers) arrive at a single delivery point (API), they get stuck waiting. This bottleneck creates a backlog, causing performance drops and delays in handling new tasks.
In a C# application, thread starvation can lead to:
-
API Request Bottlenecks: Threads waiting for permission to send requests, wasting resources.
-
Task Pile-Up: Accumulating new tasks as threads are held up.
-
Increased Latency and Reduced Throughput: Longer task completion times decrease overall throughput and user experience.
To combat this, effective concurrency management is essential. Techniques like SemaphoreSlim, Parallel.ForEachAsync, and Task.WhenAll can help mitigate these issues and maintain optimal performance under strict rate limits.
Code Explanation
Let's examine the code implementations of these techniques, focusing on their purposes, functionalities, and ideal scenarios.
-
Fast & Simple: The Power of Task.WhenAll
Purpose: Demonstrates concurrent execution using Task.WhenAll.
private Task UpsertUsingParallelForEachAsync() { var testObjects = CreateObj(); CancellationToken cancellationToken = CancellationToken.None; var tasks = testObjects.Select(x => { return _container.UpsertItemAsync(x, cancellationToken: cancellationToken); }); returnTask.WhenAll(tasks); }
Details: Initiates asynchronous upsert operations for each item in testObjects, with Task.WhenAll waiting for completion. This approach is straightforward but risks overloading resources due to simultaneous task execution.
Pros:
-
Simplicity: Easy to implement.
-
I/O-Bound Tasks: Ideal for tasks waiting on external resources.
Cons:
-
Overloading Risk: Can lead to resource strain and 429 errors.
-
No Concurrency Control: Lacks limits on concurrent tasks.
When to Use: For simple concurrent tasks or when simplicity is preferred.
When Not to Use: In rate-limited environments or when concurrency control is needed.
-
-
Controlled Concurrency: Mastering Parallel.ForEachAsync!
Purpose: Demonstrates controlled concurrency with Parallel.ForEachAsync
private Task UpsertUsingParallelForEachAsync() { var testObjects = CreateObj(); CancellationToken cancellationToken = CancellationToken.None; var maxParallism = Convert.ToInt32(Math.Ceiling((Environment.ProcessorCount * 0.75) * 2.0)); // Using 75% of the Processor with 2 Cores return Parallel.ForEachAsync(testObjects, new ParallelOptions { CancellationToken = cancellationToken, MaxDegreeOfParallelism = maxParallism }, async (record, cancellationToken) => { await _container.UpsertItemAsync(record, cancellationToken: cancellationToken); }); }
Details: Limits the number of concurrent tasks based on processor count, distributing the workload effectively.
Pros:
-
Controlled Concurrency: Prevents resource overloading.
-
Cancellation Support: Built-in support for task cancellation.
Cons:
-
Complex Setup: Requires configuring options and managing thread safety.
When to Use: When you need controlled concurrency or are handling CPU-bound tasks.
When Not to Use: For small or simple tasks.
-
-
Precision Control: SemaphoreSlim and Parallel Processing!
Purpose: Combines SemaphoreSlim with Parallel.ForEachAsync for precise concurrency control.
private Task UpsertUsingParallelForEachAsync() { var testObjects = CreateObj(); CancellationToken cancellationToken = CancellationToken.None; var semaphore = new SemaphoreSlim(2, 2); var maxParallism = Convert.ToInt32(Math.Ceiling((Environment.ProcessorCount * 0.75) * 2.0)); // Using 75% of the Processor with 2 Cores return Parallel.ForEachAsync(testObjects, new ParallelOptions { CancellationToken = cancellationToken, MaxDegreeOfParallelism = maxParallism }, async (record, cancellationToken) => { await semaphore.WaitAsync(cancellationToken); try{ await _container.UpsertItemAsync(record, cancellationToken: cancellationToken); } finally{ semaphore.Release(); } }); }
Details: This method manages concurrency while allowing parallel task processing, minimizing the risk of resource overloading.
Pros:
-
Precise Control: Manages concurrent tasks effectively.
-
Supports Resource-Constrained Environments: Reduces resource contention.
Cons:
-
Complexity: Involves semaphore management, which can be challenging.
-
Deadlocks: Incorrect usage may lead to deadlocks.
When to Use: When you need fine-grained concurrency control or are managing shared resources.
When Not to Use: In scenarios requiring high parallelism or without familiarity with synchronization.
-
Benchmarks
Performance Showdown: Which Method Reigns Supreme?
To illustrate the performance of each concurrency strategy, we conducted benchmarks comparing execution times and memory usage:
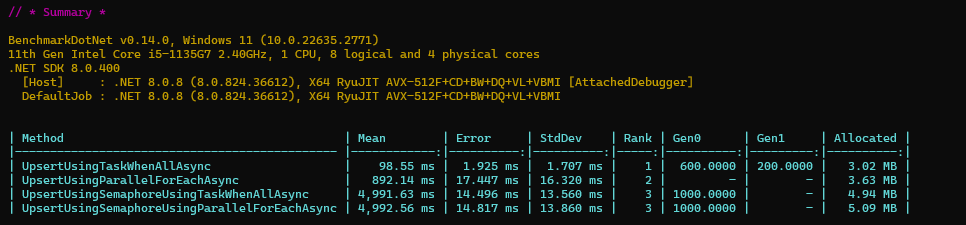
Conclusion
Choose Wisely: Unlock the Best Concurrency Strategy!
Choosing the right concurrency strategy in C# is crucial for optimizing performance. Here’s a quick recap:
-
Need for Speed? Use UpsertUsingTaskWhenAllAsync for quick task handling without rate limits.
-
Balanced Performance: Choose UpsertUsingParallelForEachAsync for a mix of speed and resource control.
-
Controlled Precision: Opt for semaphore methods for fine-grained concurrency control, keeping your app running smoothly even under pressure.
By selecting the right tool, you enhance not only performance but also reliability, ensuring your application can handle any workload effectively!